Introduction
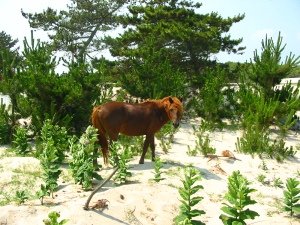
Assateague Island Pony
One of the first things we learn in any language is outputting text such as “Hello World“. Most languages support strings (unlike C’s char array) which makes storing and outputting text easy, however dealing with strings was often painful from one language to another. I will contrast between JavaFX Strings and Java Strings to help demonstrate how using them will make your pains disappear!
High level issues that many languages encounter with Strings:
- Readability – Difficult to read when string literals are large
- Expressions – Embedded expressions in strings.
- Formatting – Formatting objects into strings
Example 1 – Readability
Often folks who have worked with JEE Web apps has one time or another has hand written JDBC code using SQL statements to run against a database. And if you have used the Java Persistence API (JPA), I’m sure you have written a named query at least once. Have you ever noticed the ugliness of string concatenation?
[Disclaimer: There are plenty of object relational persistent APIs that don’t like hand built SQLs or Bean managed persistence. Just pretend you are learning JDBC for the very first time. I’m not responsible for SQL injection hacks, so please don’t use the code below in a production system.]
Here is a Java String literal containing a SQL statement:
// Java Code String param1 = "Carl"; String sql = "SELECT " + "EMP.fname, " + "EMP.lname " + "FROM " + "EMP " + "WHERE " + "EMP.fname LIKE '" + param1 + "'%";
Now let’s see how it looks in JavaFX:
// JavaFX Code def param1 = "Carl"; var sql = "SELECT " "EMP.fname," "EMP.lname " "FROM " "EMP " "WHERE " "EMP.fname LIKE '{param1}'%";
Note: You will notice the SQL statement has less clutter and the param1 expression is inside the last part of the where clause. Eazy peazy! The next example will be a more elaborate expression that is embedded in a string.
Example 2 – Expressions
// Java Code public void outputTruth(boolean news) { //String reportNews = news ? "is":"is not"; // tiny bit cryptic String reportNews = null; if (news) reportNews = "is" else reportNews = "is not"; System.out.println("The news " + reportNews + " trustworthy"); }
Let’s see the JavaFX-cellent implementation:
// JavaFX Code public function outputTruth(news:Boolean) { println("The news {if (news) "is" else "is not"} trustworthy"); }
Note: An embedded expression within the curly braces will return a string object. A good rule of thumb is to keep the expressions simple.
Example 3 – Formatting
To format an String value you must first create a format object. Remember a Java java.text.Format object is not thread safe. Synchronize it or create another one.
// Java Code Format dateFormat = new SimpleDateFormat("MM/dd/yy"); String s = formatter.format(new Date()); System.out.println(s);
Here is the JavaFX equivalent:
// JavaFX Code var s = "{%MM/dd/yy java.util.Date{}}"; println(s);
Note: After the ‘%’ is the format specified as ‘MM/dd/yy’ then a SPACE and then the object to be formatted (Date object). To see the format pattern letters take a look at the Java Doc for SimpleDateFormat.
Conclusion
Strings in JavaFX are extremely easy to use and definitely less typing. The added clarity will help people debug better. So, Happy stringing! Let me know what you think. Any feedback is always welcome.
Good overview. I was surprised the first time I saw that nested quotes were allowed inside curly braces in a string…
Another nice thing with strings in JavaFX is the simplified equality test (x == “foo” vs. x.equals(“foo”)), and the fact you can use either single or double quotes.
Auto-concatenation like in C is good too, although having HEREDOC-like syntax, like in Perl or PHP, would have been nice too.
Why on earth they don’t port at least some of these features to Java? Was it really necessary to create a new language? Do Java programmers really **like** to have less expressive language?
Marcus,
“Why on earth they don’t port at least some of these features to Java? “
-> You should ask that question on a Java forum. Seriously.
“Was it really necessary to create a new language? “
-> Sure, it was necessary. Would you use Cold fusion to build Star Craft ][? of coarse not!
Do Java programmers really **like** to have less expressive language?
No, not less expressive language, but elegantly expressed language. The main idea was to provide a DSL Domain specific language (graphics,ria domain). GUI and Graphics applications should be easier to express.
Example: Create a filled in circle with gradient in Java vs JavaFX. Which seems more natural and better expressed?
Expressing or achieving something with very few lines of code is a powerful thing. A developer should be focusing on the logic, not all the low level boiler plate details of more general purpose type languages. Many folks in the past complained about Java Swing being too hard to develop.
If you don’t see the separation of concerns and the clarity of it all, you may want to just stick to what you know.
Carl